Performance Tuning in Java Applications
- Thalles Vieira
- 12 de out. de 2024
- 3 min de leitura
A well-performing Java application is crucial in both small and large-scale environments. Performance tuning in Java focuses on several areas, including memory management, garbage collection (GC) optimization, and profiling. This article will cover these areas in detail to help developers improve the performance of their applications.
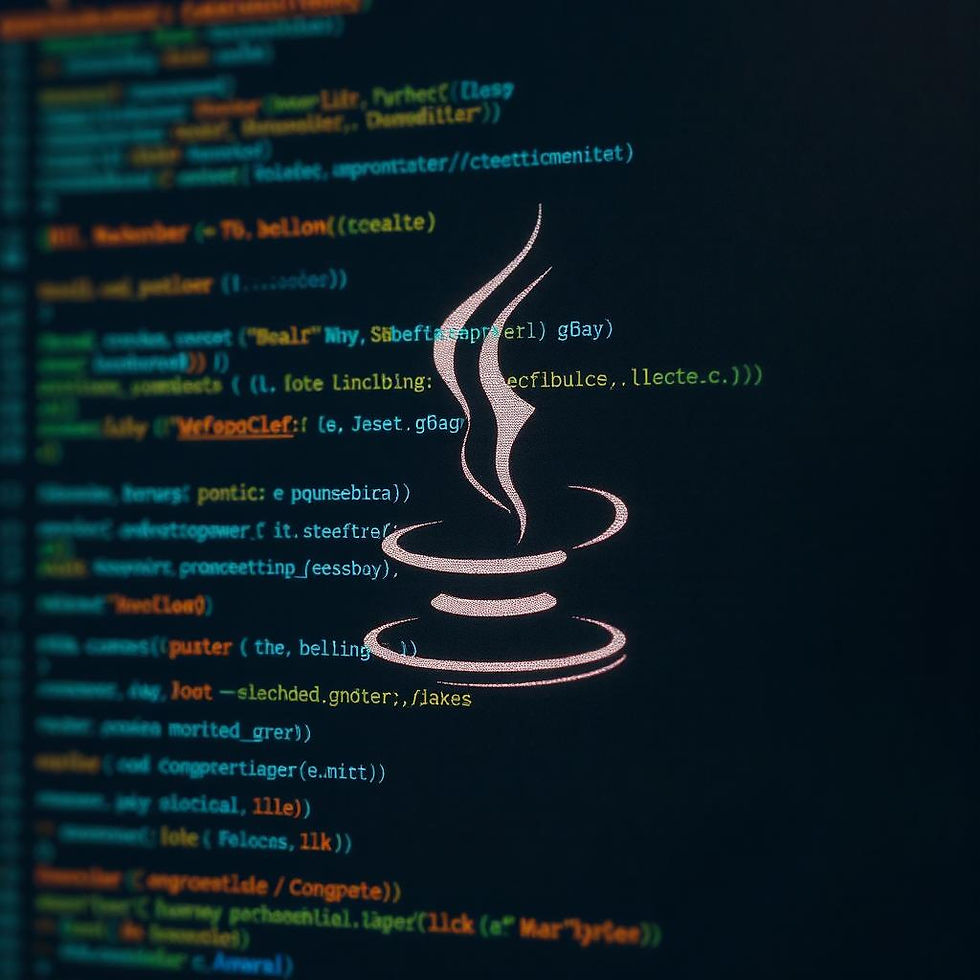
Memory Optimization
Memory optimization ensures that the application uses memory resources efficiently, avoiding issues like memory leaks, excessive garbage collection, or running out of heap space.
Heap Sizing: The JVM heap is divided into two main areas: the young generation (where new objects are allocated) and the old generation (where long-lived objects are promoted). Configuring the right heap size is critical. A small heap may lead to frequent GC cycles, while a large heap may cause long GC pauses.
Object Creation and Reuse: Excessive object creation and disposal can lead to high memory usage and frequent GC cycles. To reduce this overhead, developers can:
Reuse objects whenever possible.
Use data structures like StringBuilder instead of String concatenation in loops.
Apply the flyweight pattern for memory optimization in scenarios where objects have similar states.
Avoiding Memory Leaks: Memory leaks happen when objects are no longer needed but are still referenced, preventing GC from reclaiming the memory. Use tools like Eclipse MAT (Memory Analyzer Tool) to detect and fix memory leaks by analyzing heap dumps.
Garbage Collection (GC) Tuning
Garbage collection is the process by which the JVM reclaims memory from objects that are no longer used. Tuning the GC can have a profound effect on application performance, especially in memory-intensive applications.
Choosing the Right GC Algorithm: The JVM provides several GC algorithms that are suited for different workloads:
Serial GC: Suitable for single-threaded applications or environments with limited CPU resources.
Parallel GC: Suitable for multi-threaded applications that require high throughput but can tolerate occasional long GC pauses.
G1 GC: Targets low pause times and works well for applications that require predictable latency. It divides the heap into regions and collects them independently to minimize pause times.
ZGC and Shenandoah GC: These garbage collectors are designed for ultra-low pause times, ideal for large heap applications.
GC Tuning Parameters:
GC Pause Time Goals: With G1 GC, use -XX:MaxGCPauseMillis=<time> to define a target for the maximum pause time during garbage collection. This helps in ensuring that GC pauses don’t disrupt application performance.
Log GC Activities: Use -XX:+PrintGCDetails and -Xlog:gc to log GC activity and analyze the frequency and duration of GC pauses. By studying the logs, developers can adjust heap sizes and other GC settings.
Profiling
Profiling is the process of monitoring an application to identify performance bottlenecks. Profilers can help track CPU usage, memory consumption, thread activity, and more.
Using Profiling Tools:
JProfiler: Provides detailed insights into memory usage, CPU usage, and garbage collection activity. It helps in identifying memory leaks and pinpointing code that causes excessive object creation.
VisualVM: Bundled with the JDK, VisualVM is a lightweight tool for monitoring memory usage, CPU consumption, and thread states. It supports both real-time monitoring and heap dump analysis.
Java Flight Recorder (JFR): A low-overhead, continuous profiling tool that allows developers to record detailed JVM and application metrics over time, making it easier to diagnose performance issues in production environments.
Analyzing CPU and I/O Usage: High CPU usage may indicate that the application is performing expensive computations or that there are inefficient algorithms in use. Similarly, high I/O waits can suggest performance bottlenecks in database queries, file I/O, or network interactions. Tools like VisualVM and JProfiler can help detect these inefficiencies.
Common Java Performance Optimization Techniques
Caching: Use in-memory caching solutions like EHCache or Redis to avoid expensive computations or database queries by storing frequently accessed data in memory.
Concurrency and Threading: Use efficient concurrency patterns to avoid thread contention and reduce lock contention. Java’s java.util.concurrent package provides several utilities like ConcurrentHashMap and thread pools that can improve the performance of multi-threaded applications.
Database Optimizations: Optimize database access by:
Using connection pools (e.g., HikariCP) to reuse database connections.
Writing efficient SQL queries and indexing your database.
Using batching for insert and update operations to reduce the number of database calls.
Lazy Loading: In Hibernate and other ORM tools, enable lazy loading for entities and collections to avoid loading large amounts of data into memory unnecessarily.
Conclusion
Performance tuning in Java requires a comprehensive approach, involving memory management, garbage collection tuning, and profiling. By carefully adjusting JVM parameters, using the appropriate garbage collector, and analyzing application behavior with profiling tools, developers can significantly improve the performance of their applications across different use cases.
Comments