Exploring Kotlin Coroutines for Asynchronous Programming
- Thalles Vieira
- 11 de nov. de 2024
- 3 min de leitura
Asynchronous programming is a key technique in modern software development, enabling applications to handle long-running tasks without blocking the main thread. In Kotlin, coroutines offer an efficient, readable way to achieve this, standing out compared to traditional approaches like CompletableFuture or Thread in Java. This article delves into how Kotlin coroutines make asynchronous programming simpler and more effective, offering a comparison with Java's solutions.
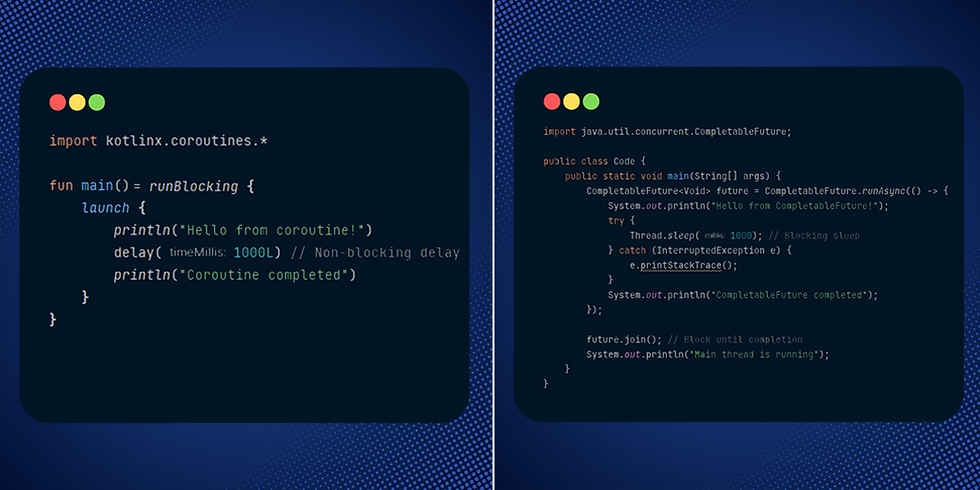
Why Kotlin Coroutines?
Kotlin coroutines were introduced to simplify the development of asynchronous, non-blocking code. Coroutines allow us to write code that is both sequential and asynchronous, avoiding the complexity of callbacks or CompletableFuture chaining common in Java. Coroutines also provide structured concurrency, helping to manage and control coroutines' lifecycle in a well-defined scope.
Here’s how they work and how they compare with alternatives in Java.
1. Basics of Coroutines
In Kotlin, coroutines are lightweight threads managed by the Kotlin runtime rather than the underlying OS. This lightweight nature allows thousands of coroutines to run concurrently, which is impractical with Java Thread.
Here’s how we can launch a coroutine in Kotlin:
In this example, launch is used to start a new coroutine that prints a message, waits for a second (without blocking), and then prints another message. The runBlocking function keeps the main thread alive until the coroutine completes.
2. Comparison with Java's CompletableFuture
Java's CompletableFuture allows developers to write asynchronous code without blocking, but it often leads to verbose and complex syntax:
While CompletableFuture provides asynchronous handling, its usage can quickly become verbose and harder to manage, especially in complex flows. Additionally, CompletableFuture relies on blocking mechanisms like Thread.sleep, which can hurt performance, unlike Kotlin’s delay, which is non-blocking.
3. Writing Efficient Asynchronous Code with Coroutines
Kotlin provides multiple coroutine builders (launch, async, runBlocking) and contexts (Dispatchers.IO, Dispatchers.Default) to handle different types of tasks efficiently. For instance, Dispatchers.IO can be used for blocking IO tasks, while Dispatchers.Default is designed for CPU-intensive tasks.
Here’s an example of using async to execute two coroutines concurrently and collect results:
In this example, both fetchUserData and fetchUserPreferences run in parallel, allowing us to collect both results in an efficient, non-blocking manner. The code remains readable and intuitive, making complex asynchronous workflows manageable.
4. Handling Exceptions in Coroutines
Another significant benefit of coroutines is the straightforward error handling with structured concurrency. If an exception occurs in one coroutine, it can propagate and affect other coroutines within the same scope, helping to handle errors more consistently. For instance:
This contrasts with CompletableFuture, where handling errors across multiple futures can become cumbersome and hard to manage.
5. Comparing Performance and Usability
Coroutines are designed to be highly performant. Each coroutine runs within a lightweight thread, consuming minimal memory and CPU resources compared to native threads in Java. As a result, Kotlin applications can handle thousands of coroutines concurrently, a feat that would be challenging with Thread or even CompletableFuture.
In addition, Kotlin’s coroutine syntax is significantly more readable. With async and await, we can achieve asynchronous programming while keeping the code linear and clean, reducing the need for complex chaining or callbacks.
Conclusion
Kotlin coroutines offer a powerful, efficient approach to asynchronous programming that improves readability, error handling, and resource management compared to traditional Java techniques. They simplify complex asynchronous workflows with a clear, structured concurrency model that makes it easier to write, debug, and maintain code.
For developers transitioning from Java, adopting Kotlin coroutines can be transformative, bringing benefits in both performance and developer productivity.
Comments